Django is a web development framework written in Python that follows the model-view-template (MVT) design pattern. Its main features are simplicity, flexibility, reliability, and scalability. Django provides all the necessary mechanisms for web programming of a site, access to databases, translations, template language, among other features. Additionally, it automatically provides a content administration interface, which allows the creation, updating, and deletion of objects, keeping a record of all actions performed, and provides an interface to manage users and user groups.
To illustrate the entire web development process in Django, we will create a project called rxWodProject, which is a training routine planning application.
This will use PostgreSQL as the database. We could start using SQLite if we wish, but the subsequent data migration can be traumatic.
We check the available versions in the FreeBSD repositories:
postgresql10-server-10.15 PostgreSQL is the most advanced open-source database available anywhere
postgresql11-server-11.10 PostgreSQL is the most advanced open-source database available anywhere
postgresql12-server-12.5 PostgreSQL is the most advanced open-source database available anywhere
postgresql13-server-13.1_1 PostgreSQL is the most advanced open-source database available anywhere
postgresql95-server-9.5.24 PostgreSQL is the most advanced open-source database available anywhere
postgresql96-server-9.6.20 PostgreSQL is the most advanced open-source database available anywhere
We install the desired version:
We add PostgreSQL to the startup:
We initialize the database:
We start the service:
By default, PostgreSQL uses the “peer authentication” authentication scheme for local connections, which basically means that if the name of an OS user matches the name of a PostgreSQL user, this user can access the PostgreSQL CLI without authentication.
psql
We create the web application access user:
CREATE USER rxwod_user WITH PASSWORD 'PASSWORD';
ALTER ROLE rxwod_user SET client_encoding TO 'utf8';
ALTER ROLE rxwod_user SET default_transaction_isolation TO 'read committed';
ALTER ROLE rxwod_user SET timezone TO 'UTC+1';
We create the database indicating the owner:
CREATE DATABASE rxwod WITH OWNER rxwod_user;
We list the databases:
\l
List of databases
Name | Owner | Encoding | Collate | Ctype | Access privileges
-----------+------------+----------+---------+---------+-----------------------
postgres | postgres | UTF8 | C | C.UTF-8 |
rxwod | rxwod_user | UTF8 | C | C.UTF-8 |
template0 | postgres | UTF8 | C | C.UTF-8 | =c/postgres +
| | | | | postgres=CTc/postgres
template1 | postgres | UTF8 | C | C.UTF-8 | =c/postgres +
| | | | | postgres=CTc/postgres
(4 rows)
We assign the necessary permissions and exit:
GRANT ALL PRIVILEGES ON DATABASE rxwod TO rxwod_user;
\q
exit
We check the access:
rxwod=> \conninfo
You are connected to database "rxwod" as user "rxwod_user" via socket in "/tmp" at port "5432".
We install Python and the basic dependencies:
We generate the virtual environment from a regular user account. If you are not familiar with Python virtual environments, I recommend
this article
:
We activate the virtual environment:
source bin/activate
While we remain in the Venv, a different prompt will appear:
(rxWod) Garrus $ ~/rxWod>
I will omit said prompt to make it easier to copy and paste the manual commands.
We install Django, SQLite, and psycopg2 inside the virtual environment:
If we have an outdated version of pip, it may ask us to update it:
WARNING: You are using pip version 20.1.1; however, version 21.0.1 is available.
You should consider upgrading via the ‘/usr/home/kr0m/rxWod/bin/python3.7 -m pip install –upgrade pip’ command.
We update it:
The pip freeze command shows us the versions of the currently installed libraries:
asgiref==3.3.1
Django==3.1.7
psycopg2==2.8.6
pytz==2021.1
sqlite3==0.0.0
sqlparse==0.4.1
Tkinter==0.0.0
We check if Django is installed:
>>> import django
>>> print(django.get_version())
3.1.7
>>> quit()
We create the project:
It will have generated the following directory structure:
|-- manage.py
`-- rxWodProject
|-- __init__.py
|-- asgi.py
|-- settings.py
|-- urls.py
`-- wsgi.py
We generate the requirements file based on the installed versions, so if someone clones our repository, they only need to install the dependencies with: pip install -r requirements.txt
pip freeze > requirements.txt
We check that the project works:
Watching for file changes with StatReloader
Performing system checks...
System check identified no issues (0 silenced).
You have 18 unapplied migration(s). Your project may not work properly until you apply the migrations for app(s): admin, auth, contenttypes, sessions.
Run 'python manage.py migrate' to apply them.
February 28, 2021 - 12:27:04
Django version 3.1.7, using settings 'rxWodProject.settings'
Starting development server at http://127.0.0.1:8000/
Quit the server with CONTROL-C.
For now, we can ignore the warning about pending migrations in the database.
We must bear in mind that this server is only for use in the testing phase, on the Django website they insist and insist that it should not be used as a production server:
Don’t use this server in anything resembling a production environment. It’s intended only for use while developing. (We’re in the business of making Web frameworks, not Web servers.)
We access the app via the web:
http://127.0.0.1:8000/
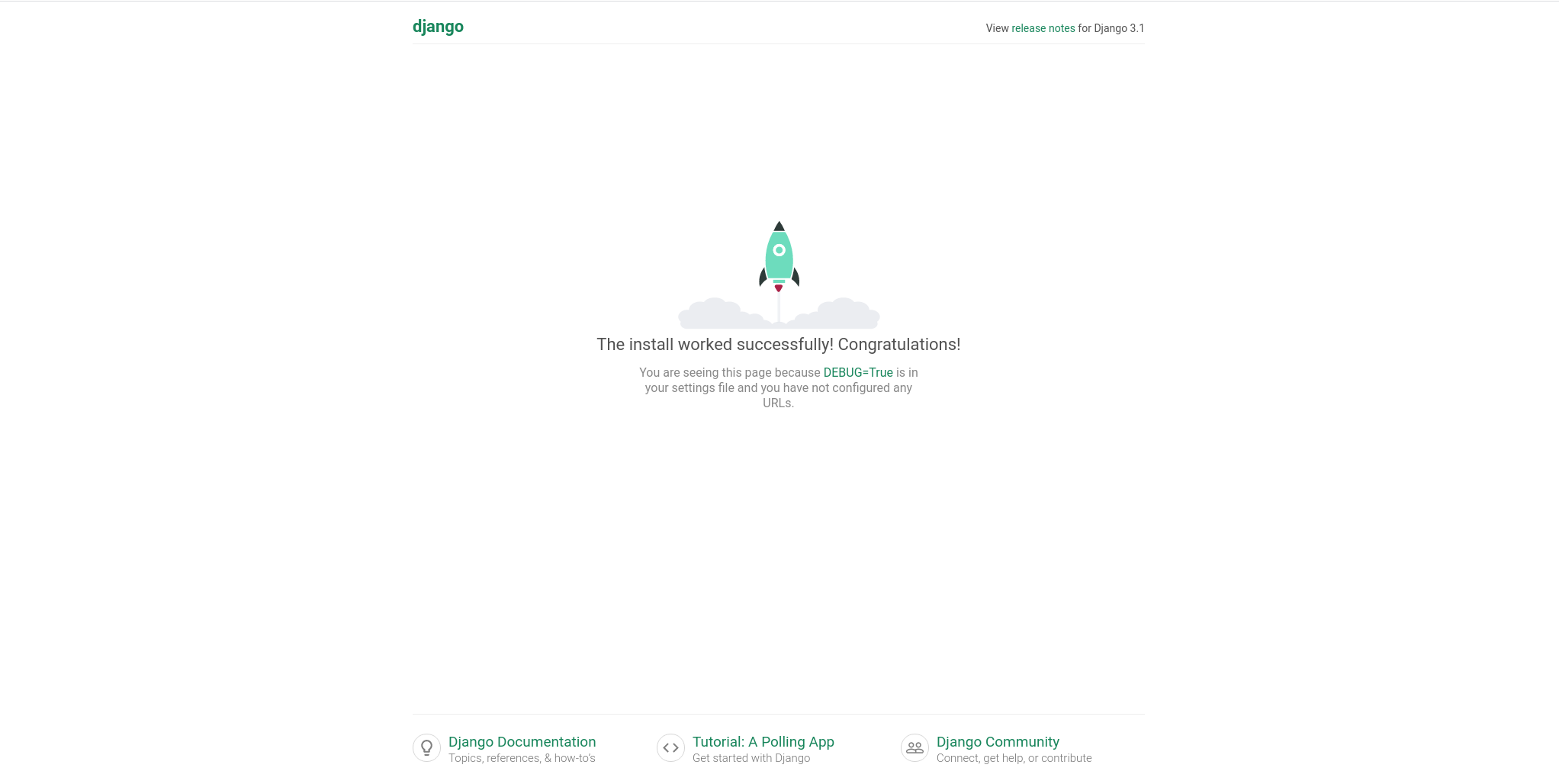
In this article, we have only scratched the surface since we have only prepared the database for later use, created a virtual environment, and created the Django project. In subsequent articles, we will create an app within the project and investigate database management.