Selenium is a software testing environment for web-based applications. It allows us to automate tasks through a browser. In this case, we will simulate a login on a website and a click on a button on that website.
We will automate this entire process using a Python script that will check the time and act accordingly. We will leave this script as a service to run indefinitely.
First, we download the Selenium version corresponding to our OS:
https://github.com/mozilla/geckodriver/releases
We move the binary to an accessible location:
We install the Python libraries to work with Selenium:
The following script will log in to the website and press the button on the days and at the hours indicated:
#!/usr/bin/python
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.desired_capabilities import DesiredCapabilities
from selenium.webdriver.firefox.options import Options
import time
import random
import schedule
import sys
from datetime import datetime
def job(executeNow):
f = open("/tmp/login.log","a")
now = datetime.now()
dt_string = now.strftime("%d/%m/%Y %H:%M:%S")
options = Options()
if executeNow == '1':
f.write("Executing job NOW: %s\r\n" % (dt_string))
else:
options.headless = True
# More human behaviour trick:
randomValue = random.randint(0,120)
time.sleep(randomValue)
f.write("Executing job: %s\r\n" % (dt_string))
f.close()
usernameStr = 'XXXXXXXXXXX'
passwordStr = 'YYYYYYYYYYY'
cap = DesiredCapabilities().FIREFOX
browser = webdriver.Firefox(options=options, capabilities=cap, executable_path="/usr/local/bin/geckodriver")
browser.get('https://URL/')
username = browser.find_element_by_id('mat-input-0')
username.send_keys(usernameStr)
password = browser.find_element_by_id('mat-input-1')
password.send_keys(passwordStr)
signInButton = browser.find_element_by_name('loginButton')
signInButton.click()
time.sleep(8)
fingerPrint = browser.find_element_by_xpath("/html/body/app-root/div/app-layout/section/div/div/div/section/app-dashboard-tasks/div/div/app-signup/div/div/div/div[2]/div/button")
fingerPrint.click()
time.sleep( 10 )
browser.quit()
if len(sys.argv) > 1:
job('1')
exit()
else:
#schedule.every(10).seconds.do(job)
#schedule.every().hour.do(job)
#schedule.every().day.at("10:30").do(job)
#schedule.every(5).to(10).minutes.do(job)
#schedule.every().monday.do(job)
#schedule.every().wednesday.at("13:15").do(job)
#schedule.every().minute.at(":17").do(job)
schedule.every().friday.at("12:41").do(job)
while True:
schedule.run_pending()
time.sleep(1)
NOTE: Almost all elements of a website can be obtained using the find_element_by_ functions of Selenium, but there are occasions when this is not possible. In these cases, we are forced to use xpaths. To obtain an xpath, we will have to right-click on the element -> Inspect, Copy -> Copy Xpath
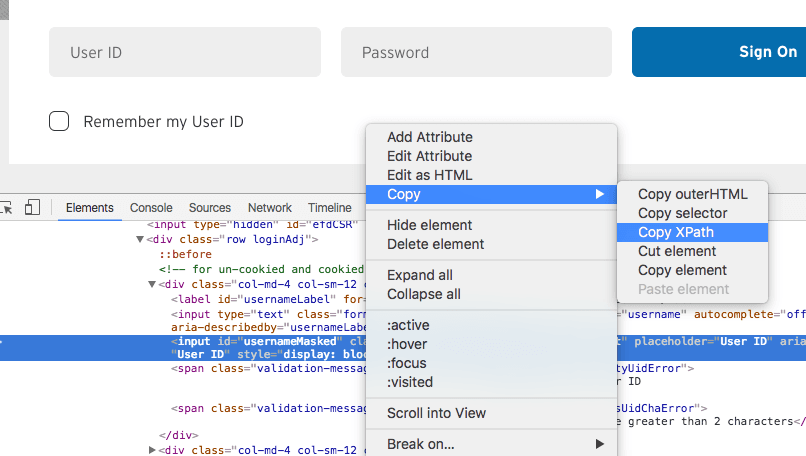
We create the service:
su kr0m -c "nohup python /home/kr0m/.scripts/login.py &"
We give it the necessary permissions:
We start the service:
We check that it is running:
kr0m 22650 0.0 0.1 88192 18396 ? S jun17 0:08 /usr/lib/python-exec/python2.7/python /home/kr0m/.scripts/login.py