OVH is one of the most important ISPs worldwide, providing all kinds of services, from servers and domains to email accounts or servers with GPUs for graphic processing. In this article, we will learn how to use its API from a Python script, so we will be able to automate many administrative tasks.
The first step will be to create an app, for which we go to:
[https://eu.api.ovh.com/createApp/]
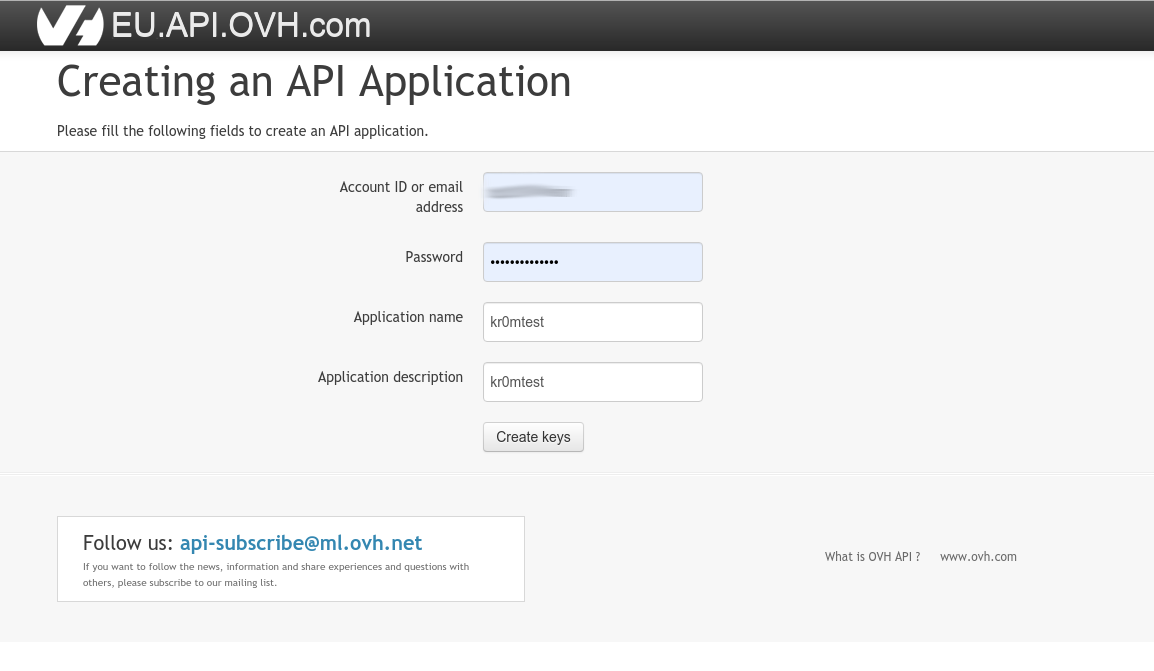
They will provide us with two parameters:
- APPLICATION_KEY
- APPLICATION_SECRET
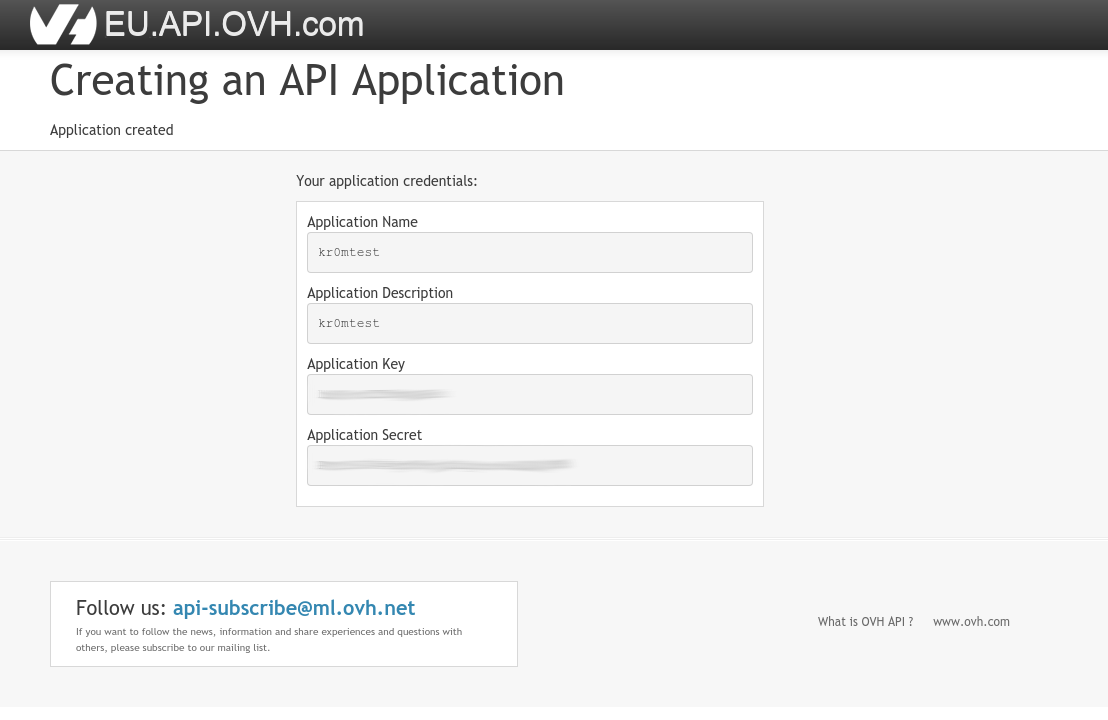
We create the configuration file with the necessary access data:
[default]
; general configuration: default endpoint
endpoint=ovh-eu
[ovh-eu]
; configuration specific to 'ovh-eu' endpoint
application_key=XXXXXXXXXXXXXX
application_secret=YYYYYYYYYYYY
;consumer_key=ZZZZZZZZZZZZ
NOTE: We leave the consumer_key commented for now.
We install the Python library to interact with the API:
We obtain the consumer_key through the following script:
import ovh
# create a client using configuration
client = ovh.Client()
# Request RO, /me API access
ck = client.new_consumer_key_request()
# Allow all GET, POST, PUT, DELETE on /* (full API)
ck.add_recursive_rules(ovh.API_READ_WRITE, '/')
# Request token
validation = ck.request()
print("Please visit %s to authenticate" % validation['validationUrl'])
input("and press Enter to continue...")
# Print nice welcome message
print("Welcome", client.get('/me')['firstname'])
print("Btw, your 'consumerKey' is '%s'" % validation['consumerKey'])
We run it:
Please visit https://eu.api.ovh.com/auth/?credentialToken=53EA76vsdfrrrrAGyN0M6HbjgGVa5qjXdYnnXsdQFowSh4ifmawon1otlGQk to authenticate
and press Enter to continue...
We visit the validationUrl and give it the validity time we need.
Now that we have the consumer_key, we edit the configuration file by uncommenting the consumer_key and assigning it the correct value:
[default]
; general configuration: default endpoint
endpoint=ovh-eu
[ovh-eu]
; configuration specific to 'ovh-eu' endpoint
application_key=XXXXXXXXXXXXXX
application_secret=YYYYYYYYYYYY
consumer_key=ZZZZZZZZZZZZ
We write a test script:
import ovh
import json
client = ovh.Client()
result = client.get('/me')
print(json.dumps(result, indent=4))
We run it:
{
"zip": "03804",
"language": "es_ES",
"firstname": "Juan Jose",
"nichandle": "XXXXXXX-ovh",
"organisation": "",
"state": "complete",
"corporationType": "",
"country": "ES",
"legalform": "individual",
"email": "kr0m@alfaexploit.com",
"city": "ALCOI",
"birthDay": "",
"name": "Ivars Poquet",
"nationalIdentificationNumber": "XXXXXXXXY",
"ovhCompany": "ovh",
"ovhSubsidiary": "ES",
"customerCode": "XXXX-YYYY-ZZ",
"area": "03",
"fax": "",
"phone": "+34.XXXYYZZWW",
"currency": {
"code": "EUR",
"symbol": "\u20ac"
},
"address": "Calle inventada 123",
"spareEmail": null,
"birthCity": "",
"companyNationalIdentificationNumber": null,
"vat": "",
"phoneCountry": "ES",
"sex": null,
"italianSDI": "0000000"
}
If we prefer, we can assign the credentials by code. This is useful if we are going to access several accounts from a single script:
import ovh
import json
APPLICATION_KEY = 'XXXXXXXXXXXXXXX'
APPLICATION_SECRET = 'YYYYYYYYYYYYYYY'
CONSUMER_KEY = 'ZZZZZZZZZZZZZZZZZZZ'
client = ovh.Client(
endpoint = 'ovh-eu',
application_key = APPLICATION_KEY,
application_secret = APPLICATION_SECRET,
consumer_key = CONSUMER_KEY,
)
result = client.get('/me')
print(json.dumps(result, indent=4))
All API calls are documented with examples included in the following URL:
[https://api.ovh.com/console/]